df_to_str_and_zip_write_str¶
In [1]:
import numpy as np
import pandas as pd
import matplotlib
import matplotlib.pyplot as plt
import seaborn as sns
%matplotlib inline
In [2]:
np.random.seed(0)
_1_to_3less = np.random.randint(1, 3, size=(7, 5))
_1_to_3less
Out[2]:
array([[1, 2, 2, 1, 2],
[2, 2, 2, 2, 2],
[2, 1, 1, 2, 1],
[1, 1, 1, 1, 2],
[1, 2, 2, 1, 1],
[2, 2, 2, 2, 1],
[2, 1, 2, 1, 2]])
In [3]:
_1_to_3 = np.random.random_integers(1, 3, size=(7, 5))
_1_to_3
Out[3]:
array([[2, 3, 1, 3, 1],
[2, 2, 3, 1, 2],
[2, 2, 1, 3, 1],
[3, 3, 1, 3, 1],
[1, 1, 2, 2, 3],
[1, 1, 2, 1, 2],
[3, 3, 1, 2, 2]])
In [4]:
df = pd.DataFrame(_1_to_3, columns=list("abcde"))
df
Out[4]:
a | b | c | d | e | |
---|---|---|---|---|---|
0 | 2 | 3 | 1 | 3 | 1 |
1 | 2 | 2 | 3 | 1 | 2 |
2 | 2 | 2 | 1 | 3 | 1 |
3 | 3 | 3 | 1 | 3 | 1 |
4 | 1 | 1 | 2 | 2 | 3 |
5 | 1 | 1 | 2 | 1 | 2 |
6 | 3 | 3 | 1 | 2 | 2 |
In [5]:
print(df.to_string(index=False, header=False), end="")
2 3 1 3 1
2 2 3 1 2
2 2 1 3 1
3 3 1 3 1
1 1 2 2 3
1 1 2 1 2
3 3 1 2 2
In [6]:
import io
strio = io.StringIO()
_str = df.to_string(buf=strio)
print(_str)
print(strio.getvalue())
None
a b c d e
0 2 3 1 3 1
1 2 2 3 1 2
2 2 2 1 3 1
3 3 3 1 3 1
4 1 1 2 2 3
5 1 1 2 1 2
6 3 3 1 2 2
In [39]:
strio = io.StringIO()
df.to_string(buf=strio)
for l in strio:
print(l)
In [40]:
strio = io.StringIO(df.to_string())
for l in strio:
print(l)
a b c d e
0 2 3 1 3 1
1 2 2 3 1 2
2 2 2 1 3 1
3 3 3 1 3 1
4 1 1 2 2 3
5 1 1 2 1 2
6 3 3 1 2 2
In [72]:
import zipfile
from contextlib import ExitStack
with ExitStack() as s:
_bio = s.enter_context(io.BytesIO())
z = s.enter_context(zipfile.ZipFile(_bio, "w", compression=zipfile.ZIP_DEFLATED))
z.writestr("a.txt", "aaaa")
z.writestr("c/d.txt", "ccdd")
print(_bio.getvalue()[:4])
b'PK\x03\x04'
In [65]:
import zipfile
_zio = io.StringIO()
with zipfile.ZipFile(_zio, "w", compression=zipfile.ZIP_DEFLATED) as z:
z.writestr("a.txt", "aaaa")
# z.writestr("a.txt", bytes("aaaa"))
# z.writestr("b.txt", "bbb".encode())
# z.writestr("c/d.txt", "ccdd")
_zio.getvalue()[:4]
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
<ipython-input-65-112a2cb7597a> in <module>()
4 with zipfile.ZipFile(_zio, "w", compression=zipfile.ZIP_DEFLATED) as z:
----> 5 z.writestr("a.txt", "aaaa")
6 # z.writestr("a.txt", bytes("aaaa"))
/Users/knt/.pyenv/versions/anaconda3-2.5.0/lib/python3.5/zipfile.py in writestr(self, zinfo_or_arcname, data, compress_type)
1578 raise LargeZipFile("Filesize would require ZIP64 extensions")
-> 1579 self.fp.write(zinfo.FileHeader(zip64))
1580 self.fp.write(data)
TypeError: string argument expected, got 'bytes'
During handling of the above exception, another exception occurred:
TypeError Traceback (most recent call last)
<ipython-input-65-112a2cb7597a> in <module>()
3 _zio = io.StringIO()
4 with zipfile.ZipFile(_zio, "w", compression=zipfile.ZIP_DEFLATED) as z:
----> 5 z.writestr("a.txt", "aaaa")
6 # z.writestr("a.txt", bytes("aaaa"))
7 # z.writestr("b.txt", "bbb".encode())
/Users/knt/.pyenv/versions/anaconda3-2.5.0/lib/python3.5/zipfile.py in __exit__(self, type, value, traceback)
1067
1068 def __exit__(self, type, value, traceback):
-> 1069 self.close()
1070
1071 def __repr__(self):
/Users/knt/.pyenv/versions/anaconda3-2.5.0/lib/python3.5/zipfile.py in close(self)
1604 if self._seekable:
1605 self.fp.seek(self.start_dir)
-> 1606 self._write_end_record()
1607 finally:
1608 fp = self.fp
/Users/knt/.pyenv/versions/anaconda3-2.5.0/lib/python3.5/zipfile.py in _write_end_record(self)
1707 0, 0, centDirCount, centDirCount,
1708 centDirSize, centDirOffset, len(self._comment))
-> 1709 self.fp.write(endrec)
1710 self.fp.write(self._comment)
1711 self.fp.flush()
TypeError: string argument expected, got 'bytes'
In [48]:
import zipfile
with zipfile.ZipFile("file.zip", "w", zipfile.ZIP_DEFLATED) as z:
z.writestr("file.txt", df.to_string())
In [42]:
import os
now = pd.to_datetime("now").date().isoformat()
df.to_csv(now, index=False, header=False)
with open(now) as f:
print(f.read())
os.remove(now)
2,3,1,3,1
2,2,3,1,2
2,2,1,3,1
3,3,1,3,1
1,1,2,2,3
1,1,2,1,2
3,3,1,2,2
In [43]:
sns.pairplot(data=df, hue="d")
Out[43]:
<seaborn.axisgrid.PairGrid at 0x12094fc88>
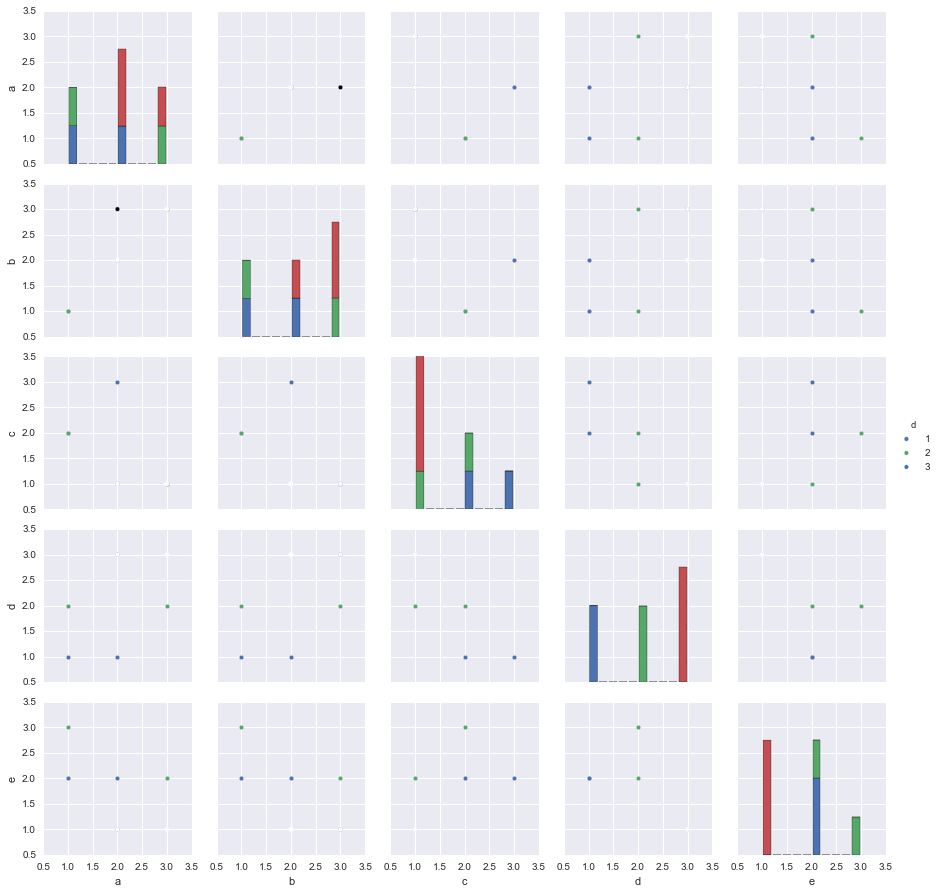